Constructor Chaining In Java with Examples
Last Updated :
04 Jul, 2022
Constructor chaining is the process of calling one constructor from another constructor with respect to current object.
One of the main use of constructor chaining is to avoid duplicate codes while having multiple constructor (by means of constructor overloading) and make code more readable.
Prerequisite - Constructors in Java
Constructor chaining can be done in two ways:
- Within same class: It can be done using this() keyword for constructors in the same class
- From base class: by using super() keyword to call the constructor from the base class.
Constructor chaining occurs through inheritance. A sub-class constructor’s task is to call super class’s constructor first. This ensures that the creation of sub class’s object starts with the initialization of the data members of the superclass. There could be any number of classes in the inheritance chain. Every constructor calls up the chain till the class at the top is reached.
Why do we need constructor chaining?
This process is used when we want to perform multiple tasks in a single constructor rather than creating a code for each task in a single constructor we create a separate constructor for each task and make their chain which makes the program more readable.
Constructor Chaining within the same class using this() keyword:
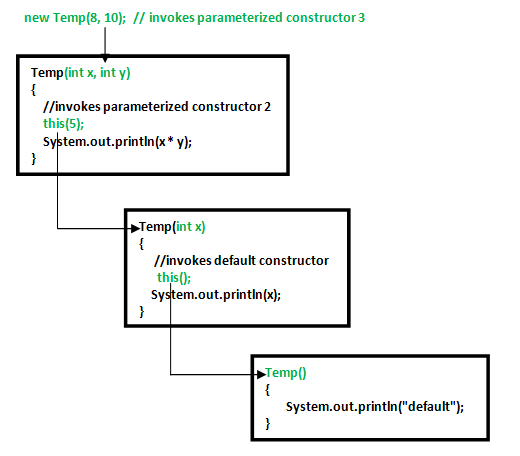
Java
class Temp
{
Temp()
{
this ( 5 );
System.out.println( "The Default constructor" );
}
Temp( int x)
{
this ( 5 , 15 );
System.out.println(x);
}
Temp( int x, int y)
{
System.out.println(x * y);
}
public static void main(String args[])
{
new Temp();
}
}
|
Output:
75
5
The Default constructor
Rules of constructor chaining :
- The this() expression should always be the first line of the constructor.
- There should be at-least be one constructor without the this() keyword (constructor 3 in above example).
- Constructor chaining can be achieved in any order.
What happens if we change the order of constructors?
Nothing, Constructor chaining can be achieved in any order
Java
class Temp
{
Temp()
{
System.out.println( "default" );
}
Temp( int x)
{
this ();
System.out.println(x);
}
Temp( int x, int y)
{
this ( 5 );
System.out.println(x * y);
}
public static void main(String args[])
{
new Temp( 8 , 10 );
}
}
|
Output:
default
5
80
NOTE: In example 1, default constructor is invoked at the end, but in example 2 default constructor is invoked at first. Hence, order in constructor chaining is not important.
Constructor Chaining to other class using super() keyword :
Java
class Base
{
String name;
Base()
{
this ( "" );
System.out.println( "No-argument constructor of" +
" base class" );
}
Base(String name)
{
this .name = name;
System.out.println( "Calling parameterized constructor"
+ " of base" );
}
}
class Derived extends Base
{
Derived()
{
System.out.println( "No-argument constructor " +
"of derived" );
}
Derived(String name)
{
super (name);
System.out.println( "Calling parameterized " +
"constructor of derived" );
}
public static void main(String args[])
{
Derived obj = new Derived( "test" );
}
}
|
Output:
Calling parameterized constructor of base
Calling parameterized constructor of derived
Note : Similar to constructor chaining in same class, super() should be the first line of the constructor as super class’s constructor are invoked before the sub class’s constructor.
Alternative method : using Init block :
When we want certain common resources to be executed with every constructor we can put the code in the init block. Init block is always executed before any constructor, whenever a constructor is used for creating a new object.
Example 1:
Java
class Temp
{
{
System.out.println( "init block" );
}
Temp()
{
System.out.println( "default" );
}
Temp( int x)
{
System.out.println(x);
}
public static void main(String[] args)
{
new Temp();
new Temp( 10 );
}
}
|
Output:
init block
default
init block
10
NOTE: If there are more than one blocks, they are executed in the order in which they are defined within the same class. See the ex.
Example :
Java
class Temp
{
{
System.out.println( "init" );
}
Temp()
{
System.out.println( "default" );
}
Temp( int x)
{
System.out.println(x);
}
{
System.out.println( "second" );
}
public static void main(String args[])
{
new Temp();
new Temp( 10 );
}
}
|
Output :
init
second
default
init
second
10