Go Tutorial
Last Updated :
04 Feb, 2025
Improve
Go or you say Golang is a procedural and statically typed programming language having the syntax similar to C programming language. It was developed in 2007 by Robert Griesemer, Rob Pike, and Ken Thompson at Google but launched in 2009 as an open-source programming language and mainly used in Google’s production systems. Golang is one of the most trending programming languages among developers. It provides a rich standard library, garbage collection, and dynamic-typing capability.
Overview
- Introduction to Golang
- Installing Golang on Windows
- Installing Golang on MacOS
- Hello World! in Golang
- Golang vs C++
- Golang vs Java
- Golang vs Python
Fundamentals
- Identifiers in Golang
- Keywords in Golang
- Data Types
- Variables
- Constants
- Rune in Golang
- Operators in Golang
- Scope of Variables
- Type Casting
- var Keyword in Golang
- Short Declaration Operator(:=)
- var keyword vs short declaration operator
Control Statements
- Decision Making Statements
- Loops in Golang
- Loop Control Statements
- Switch Statement in Go
- Deadlock and Default Case in Select Statement
Functions & Methods
- What are the Functions?
- Variadic Function
- Anonymous Function
- main and init function
- Function Arguments
- Function Returning Multiple Values
- Named Return Values
- Blank Identifier
- Defer
- Methods
- Methods With Same Name
Structure
- Structures
- Structure Equality
- Nested Structure
- Anonymous Structure and Fields
- Promoted Fields in Structure
- Promoted Methods in Structure
- Function as a Field in Structure
Arrays & Slices
- Arrays in Golang
- Copying an Array into Another Array in Golang
- Passing an Array to a Function in Golang
- Slices in Golang
- Slice Composite Literal
- Copying one Slice into another Slice
- Passing a Slice to Function
- Comparing two Slices in Golang
- Checking the Equality of Slices in Golang
- Sorting a Slice in Golang
- Trimming a Slice in Golang
- Splitting a Slice in Golang
String
- Strings in Golang
- Different ways to compare Strings
- Different ways to concatenate two strings
- Trimming a String in Golang
- Splitting a String in Golang
- Check if the given characters are present in String
- Repeating a String for Specific Number of Times
- Finding the index value of specified string
- Counting the Number of Repeated Characters in String
Pointers
- Pointers
- Pointer to Pointer (Double Pointer)
- Pointers to a Function
- Returning Pointer from a Function
- Pointer to an Array as Function Argument
- Pointer to Struct
- Comparing Pointers
- Finding the Capacity of the Pointer
- Finding the Length of the Pointer
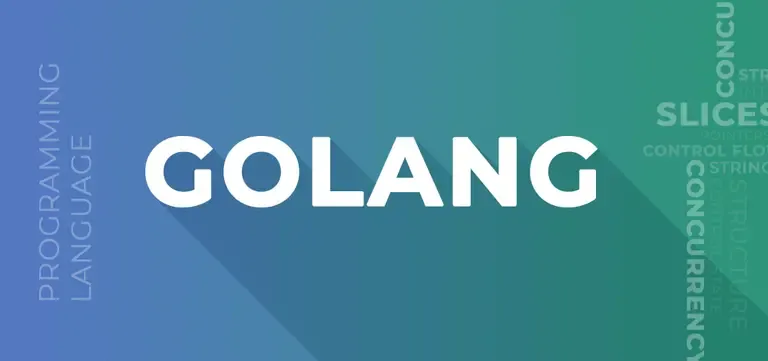
Interfaces
Concurrency
- Goroutines – Concurrency
- Select Statement
- Multiple Goroutines
- Goroutine vs Thread
- Channel in Golang
- Unidirectional Channel in Golang