Java if-else
Last Updated :
02 Apr, 2024
Decision-making in Java helps to write decision-driven statements and execute a particular set of code based on certain conditions. The if statement alone tells us that if a condition is true it will execute a block of statements and if the condition is false it won’t. In this article, we will learn about Java if-else.
If-Else in Java
If- else together represents the set of Conditional statements in Java that are executed according to the condition which is true.
Syntax of if-else Statement
if (condition)
{
// Executes this block if
// condition is true
}
else
{
// Executes this block if
// condition is false
}
Java if-else Flowchart
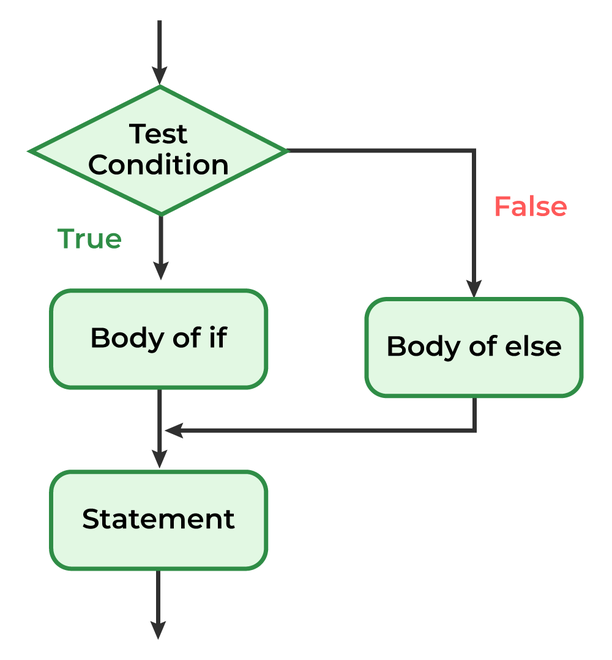
if-else Program in Java
Dry-Run of if-else statements
1. Program starts.
2. i is initialized to 20.
3. if-condition is checked. 20<15, yields false.
4. flow enters the else block.
4.a) "i is greater than 15" is printed
5. "Outside if-else block" is printed.
Below is the implementation of the above statements:
Java
// Java program to illustrate if-else statement
class IfElseDemo {
public static void main(String args[])
{
int i = 20;
if (i < 15)
System.out.println("i is smaller than 15");
else
System.out.println("i is greater than 15");
System.out.println("Outside if-else block");
}
}
Outputi is greater than 15
Outside if-else block
Nested if statement in Java
In Java, we can use nested if statements to create more complex conditional logic. Nested if statements are if statements inside other if statements.
Syntax:
if (condition1) {
// code block 1
if (condition2) {
// code block 2
}
}
Below is the implementation of Nested if statements:
Java
// Java Program to implementation
// of Nested if statements
// Driver Class
public class AgeWeightExample {
// main function
public static void main(String[] args) {
int age = 25;
double weight = 65.5;
if (age >= 18) {
if (weight >= 50.0) {
System.out.println("You are eligible to donate blood.");
} else {
System.out.println("You must weigh at least 50 kilograms to donate blood.");
}
} else {
System.out.println("You must be at least 18 years old to donate blood.");
}
}
}
OutputYou are eligible to donate blood.
Note: The first print statement is in a block of “if” so the second statement is not in the block of “if”. The third print statement is in else but that else doesn’t have any corresponding “if”. That means an “else” statement cannot exist without an “if” statement.
Frequently Asked Questions
What is the if-else in Java?
If-else is conditional statements that execute according to the correct statement executed. If the if condition is true then the code block inside the if statement is executed else it executes the else statement block.
What is the syntax for if-else?
The syntax for if-else:
if (condition)
{
// Executes this block if
// condition is true
}
else
{
// Executes this block if
// condition is false
}
Please Login to comment...