jQuery is a lightweight JavaScript library that simplifies the HTML DOM manipulating, event handling, and creating dynamic web experiences. The main purpose of jQuery is to simplify the usage of JavaScript on websites. jQuery achieves this by providing concise, single-line methods for complex JavaScript tasks, making your code more readable and maintainable. By learning jQuery, you can significantly enhance the interactivity and responsiveness of your web pages. In this jQuery tutorial, you will learn all the basic to advanced concepts of jQuery such as DOM manipulation and event handling, AJAX, animations, plugins, etc.
What is jQuery?
jQuery is a lightweight, “write less, do more” JavaScript library that simplifies web development. It provides an easy-to-use API for common tasks, making it much easier to work with JavaScript on your website. It streamlines web development by providing a concise syntax and powerful utilities for creating interactive and dynamic web pages.
Features of jQuery
Here are some key points about jQuery:
- DOM Manipulation: jQuery simplifies HTML DOM tree traversal and manipulation. You can easily select and modify elements on your web page.
- Event Handling: Handling user interactions (such as clicks or mouse events) becomes straightforward with jQuery.
- CSS Animations: You can create smooth animations and effects using jQuery.
- AJAX (Asynchronous JavaScript and XML): jQuery simplifies making asynchronous requests to the server and handling responses.
- Cross-Browser Compatibility: jQuery abstracts browser-specific inconsistencies, ensuring your code works consistently across different browsers.
- Community Support: A large community of developers actively contributes to jQuery, ensuring continuous updates and improvements.
- Plugins: jQuery has a wide range of plugins available for various tasks.
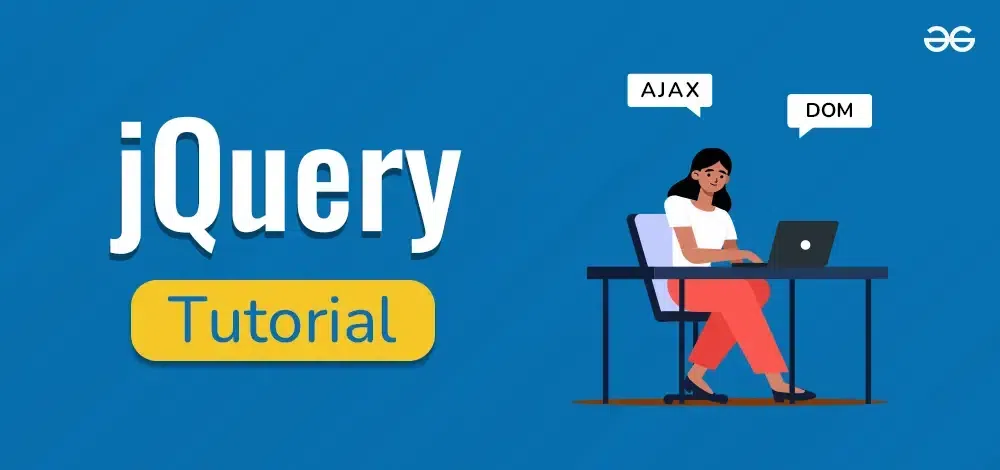
Getting Started with jQuery
1. Download the jQuery Liberary
You can download the jQuery library from the official website jquery.com and include it in your project by linking to it using a <script> tag, and host it on your server or local filesystem.
2. Include the jQuery CDN in Code
Using jQuery Library Content Delivery Network (CDN) in your HTML project.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
Once the library is included, you can write jQuery code within <script>
tags. jQuery code typically follows a specific syntax:
<script> $(document).ready(function() { // Your jQuery code here });</script>
The $(document).ready(function() { ... })
function ensures your code executes only after the document is fully loaded, preventing errors.
jQuery Basic Example
In this example, we are using hover() and css() methods to change the style of heading content on mouse move over.
html
<!DOCTYPE html>
<html>
<head>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script>
$(document).ready(function () {
$("h1").hover(
function () {
$(this).css(
"color",
"green"
);
},
function () {
$(this).css(
"color",
"aliceblue"
);
}
);
});
</script>
</head>
<body>
<h1>GeeksforGeeks</h1>
</body>
</html>
Output:
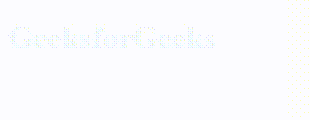
Steps to Learn jQuery – Complete jQuery Learning Path
jQuery Basics
jQuery Basics covers the fundamental concepts of the jQuery library, including its purpose, installation, and basic syntax. It introduces how to include jQuery in a web page and use its core functions to simplify JavaScript operations. Understanding the basics provides a foundation for using jQuery effectively to manipulate HTML, handle events, and create interactive web experiences.
jQuery Selectors
jQuery Selectors are used to select and manipulate HTML elements based on their attributes, classes, IDs, or other criteria. jQuery offers a powerful set of selectors that allow developers to target elements efficiently. Common selectors include ID selectors (#id), class selectors (.class), and attribute selectors ([attribute=value]).
For a comprehensive list of Selectors, please refer to the jQuery Selectors Complete Reference article.
jQuery Events
jQuery Events involve handling user interactions and other occurrences on a web page. jQuery provides methods to attach event handlers to elements, such as clicks, keypresses, and form submissions. Key functions include .on(), .off(), and .trigger(). Understanding how to work with events is essential for creating responsive and interactive web applications.
For a comprehensive list of Events, please refer to the jQuery Events Complete Reference article.
jQuery Effects
jQuery Effects enable the creation of animations and visual effects on web pages. jQuery includes built-in methods for showing, hiding, fading, sliding, and animating elements. Functions like .fadeIn(), .slideUp(), and .animate() help in creating dynamic and engaging user interfaces. Effects can enhance the user experience by providing visual feedback and transitions.
For a comprehensive list of Effects, please refer to the jQuery Effects Complete Reference article.
jQuery HTML/CSS
jQuery HTML/CSS focuses on manipulating the HTML content and CSS styles of elements using jQuery. This includes methods for changing element attributes, updating text or HTML content, and applying or modifying CSS styles. Functions such as .html(), .css(), and .addClass() allow developers to dynamically adjust the appearance and content of web elements.
For a comprehensive list of HTML/CSS, please refer to the jQuery HTML/CSS Complete Reference article.
jQuery Traversing
jQuery Traversing involves navigating the DOM tree to select and manipulate elements relative to each other. jQuery provides methods for moving up, down, and across the DOM hierarchy, such as .parent(), .children(), .siblings(), and .find(). Effective traversing helps in targeting specific elements based on their relationships within the document structure.
For a comprehensive list of Traversing, please refer to the jQuery Traversing Complete Reference article.
jQuery Ajax
jQuery Ajax facilitates asynchronous communication between the client and server, allowing web pages to update content dynamically without a full page reload. jQuery’s .ajax(), .get(), and .post() methods simplify making HTTP requests and handling responses. Understanding Ajax is essential for creating modern, responsive web applications that interact with server-side resources.
For a comprehensive list of Ajax, please refer to the jQuery Ajax Complete Reference article.
jQuery Properties
jQuery Properties cover methods for retrieving and setting properties of HTML elements. This includes getting or setting values, attributes, and other properties like dimensions and scroll positions. Functions such as .val(), .attr(), and .prop() enable precise control over element properties and are crucial for form handling and dynamic content updates.
jQuery Plugins
jQuery Plugins are extensions that add functionality to the core jQuery library. Plugins provide reusable components and features that can be easily integrated into web projects. Popular plugins include sliders, date pickers, and form validation tools. Understanding how to use and create plugins can significantly enhance the capabilities of jQuery and streamline development processes.
For a comprehensive list of Plugins, please refer to the jQuery Plugins Complete Reference article.
jQuery Projects
jQuery projects leverage the library’s features to create interactive and dynamic web applications. They often include functionalities like form validation, animations, and AJAX requests. By utilizing jQuery’s simple syntax and cross-browser compatibility, developers can efficiently build responsive and user-friendly interfaces, enhancing the overall user experience and streamlining development processes.
jQuery Interview Questions and Answers (2024)
Advantages of jQuery
- Wide range of plug-ins that allows developers to create plug-ins on top of the JavaScript library.
- Large development community.
- It is a lot easier to use compared to standard javascript and other javascript libraries.
- It lets users develop Ajax templates with ease. Ajax enables a sleeker interface where actions can be performed on pages without requiring the entire page to be reloaded.
- Being Light weight and a powerful chaining capabilities makes it more strong.
jQuery Cheat Sheet
The jQuery Cheat Sheet provides a quick reference guide for developers, summarizing common jQuery methods, selectors, events, and syntax, making it easier to write and understand jQuery code efficiently.
jQuery Tutorial – FAQs
Why should I use jQuery?
jQuery simplifies JavaScript coding by providing easy-to-use methods for common tasks, such as manipulating the DOM, handling events, and making AJAX requests. It enhances productivity and ensures compatibility across different web browsers.
How do I include jQuery in my project?
You can include jQuery in your project by adding a <script> tag in your HTML file. You can either use a Content Delivery Network (CDN) or download and host the jQuery file locally. Example for CDN: <script src=”https://code.jquery.com/jquery-3.6.0.min.js”></script>.
What are jQuery selectors?
jQuery selectors are used to find and manipulate HTML elements on a web page. They follow a similar syntax to CSS selectors, allowing you to select elements by tag, class, id, attribute, and more.
How can I handle events with jQuery?
jQuery simplifies event handling with methods like .on(), .click(), .hover(), and .submit(). You can attach event handlers to elements and define the actions that should occur when an event is triggered.
How can I traverse the DOM with jQuery?
jQuery offers various methods to traverse the DOM, such as .parent(), .children(), .siblings(), and .find(). These methods help you navigate and manipulate the hierarchy of HTML elements.
What are jQuery plugins and how do I use them?
jQuery plugins are reusable code extensions that provide additional functionality. To use a plugin, you include the plugin’s JavaScript file and initialize it on your jQuery objects using the plugin’s specific method.