Types of Statements in JDBC
Last Updated :
07 Aug, 2024
The Statement
interface in JDBC is used to create SQL statements in Java and execute queries with the database. There are different types of statements used in JDBC:
- Create Statement
- Prepared Statement
- Callable Statement
1. Â Create a Statement:
A Statement
object is used for general-purpose access to databases and is useful for executing static SQL statements at runtime.
Syntax:
Statement statement = connection.createStatement();
Implementation: Once the Statement object is created, there are three ways to execute it.
- boolean execute(String SQL): If the ResultSet object is retrieved, then it returns true else false is returned. Is used to execute SQL DDL statements or for dynamic SQL.
- int executeUpdate(String SQL): Returns number of rows that are affected by the execution of the statement, used when you need a number for INSERT, DELETE or UPDATE statements.
- ResultSet executeQuery(String SQL): Returns a ResultSet object. Used similarly as SELECT is used in SQL.
Example:
Java
// Java Program illustrating Create Statement in JDBC
// Importing Database(SQL) classes
import java.sql.*;
// Class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Try block to check if any exceptions occur
try {
// Step 2: Loading and registering drivers
// Loading driver using forName() method
Class.forName("com.mysql.cj.jdbc.Driver");
// Registering driver using DriverManager
Connection con = DriverManager.getConnection(
"jdbc:mysql:///world", "root", "12345");
// Step 3: Create a statement
Statement statement = con.createStatement();
String sql = "select * from people";
// Step 4: Execute the query
ResultSet result = statement.executeQuery(sql);
// Step 5: Process the results
// Condition check using hasNext() method which
// holds true till there is single element
// remaining in List
while (result.next()) {
// Print name an age
System.out.println(
"Name: " + result.getString("name"));
System.out.println(
"Age:" + result.getString("age"));
}
}
// Catching database exceptions if any
catch (SQLException e) {
// Print the exception
System.out.println(e);
}
// Catching generic ClassNotFoundException if any
catch (ClassNotFoundException e) {
// Print and display the line number
// where exception occurred
e.printStackTrace();
}
}
}
Output:
Name and age are as shown for random inputs.
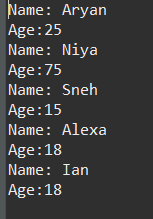
2. Prepared Statement:
A PreparedStatement
represents a precompiled SQL statement that can be executed multiple times. It accepts parameterized SQL queries, with ?
as placeholders for parameters, which can be set dynamically.
Illustration:Â
Considering in the people database if there is a need to INSERT some values, SQL statements such as these are used:Â
INSERT INTO people VALUES ("Ayan",25);
INSERT INTO people VALUES("Kriya",32);
To do the same in Java, one may use Prepared Statements and set the values in the ? holders, setXXX() of a prepared statement is used as shown:Â
String query = "INSERT INTO people(name, age)VALUES(?, ?)";
PreparedStatement pstmt = con.prepareStatement(query);
pstmt.setString(1,"Ayan");
ptstmt.setInt(2,25);
// where pstmt is an object name
Implementation: Once the PreparedStatement object is created, there are three ways to execute it:Â
- execute(): This returns a boolean value and executes a static SQL statement that is present in the prepared statement object.
- executeQuery(): Returns a ResultSet from the current prepared statement.
- executeUpdate(): Returns the number of rows affected by the DML statements such as INSERT, DELETE, and more that is present in the current Prepared Statement.
Example:Â
Java
// Java Program illustrating Prepared Statement in JDBC
// Step 1: Importing DB(SQL here) classes
import java.sql.*;
// Importing Scanner class to
// take input from the user
import java.util.Scanner;
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// try block to check for exceptions
try {
// Step 2: Establish a connection
// Step 3: Load and register drivers
// Loading drivers using forName() method
Class.forName("com.mysql.cj.jdbc.Driver");
// Scanner class to take input from user
Scanner sc = new Scanner(System.in);
// Display message for ease for user
System.out.println(
"What age do you want to search?? ");
// Reading age an primitive datatype from user
// using nextInt() method
int age = sc.nextInt();
// Registering drivers using DriverManager
Connection con = DriverManager.getConnection(
"jdbc:mysql:///world", "root", "12345");
// Step 4: Create a statement
PreparedStatement ps = con.prepareStatement(
"select name from world.people where age = ?");
// Step 5: Execute the query
ps.setInt(1, age);
ResultSet result = ps.executeQuery();
// Step 6: Process the results
// Condition check using next() method
// to check for element
while (result.next()) {
// Print and display elements(Names)
System.out.println("Name : "
+ result.getString(1));
}
// Step 7: Closing the connections
// (Optional but it is recommended to do so)
}
// Catch block to handle database exceptions
catch (SQLException e) {
// Display the DB exception if any
System.out.println(e);
}
// Catch block to handle class exceptions
catch (ClassNotFoundException e) {
// Print the line number where exception occurred
// using printStackTrace() method if any
e.printStackTrace();
}
}
}
Output:Â
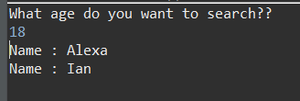
3. Callable Statement:
A CallableStatement
is used to execute stored procedures in the database. Stored procedures are precompiled SQL statements that can be called with parameters. They are useful for executing complex operations that involve multiple SQL statements.
Syntax: To create a CallableStatement,
CallableStatement cstmt = con.prepareCall("{call ProcedureName(?, ?)}");
{call ProcedureName(?, ?)}:
Calls a stored procedure named ProcedureName
with placeholders ?
for input parameters.
Methods to Execute:
- execute(): Executes the stored procedure and returns a boolean indicating whether the result is a
ResultSet
(true) or an update count (false). - executeQuery(): Executes a stored procedure that returns a
ResultSet
. - executeUpdate(): Executes a stored procedure that performs an update and returns the number of rows affected.
Example:
Java
// Java Program illustrating Callable Statement in JDBC
// Importing DB(SQL) classes
import java.sql.*;
public class GFG {
// Main driver method
public static void main(String[] args) {
// Try block to check if any exceptions occur
try {
// Step 1: Load and register the driver
Class.forName("com.mysql.cj.jdbc.Driver");
// Step 2: Establish a connection
Connection con = DriverManager.getConnection("jdbc:mysql:///world", "root", "12345");
// Step 3: Create a CallableStatement
CallableStatement cs = con.prepareCall("{call GetPeopleInfo()}");
// Step 4: Execute the stored procedure
ResultSet result = cs.executeQuery();
// Step 5: Process the results
while (result.next()) {
// Print and display elements (Name and Age)
System.out.println("Name : " + result.getString("name"));
System.out.println("Age : " + result.getInt("age"));
}
// Step 6: Close resources
result.close();
cs.close();
con.close();
}
// Catch block for SQL exceptions
catch (SQLException e) {
e.printStackTrace();
}
// Catch block for ClassNotFoundException
catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Output:Â
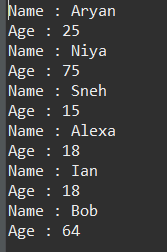
Explanation of the Program:
- This Java code demonstrates how to use a
CallableStatement
in JDBC to execute a stored procedure. - It connects to a MySQL database and prepares a
CallableStatement
to call a stored procedure named peopleinfo
with two parameters. - After executing the procedure, it runs a
SELECT
query to retrieve and display all records from the people
table. - Exception handling is included to manage potential SQL and class loading errors.
Please Login to comment...