Manual:Hooks: Difference between revisions
→Alphabetical list of hooks: part 2 because i'm dumb |
→Alphabetical list of hooks: correct name ... |
||
Line 792: | Line 792: | ||
| <span style="display:none">1.120</span>1.12.0 || [[Special:MyLanguage/Manual:Hooks/ChangesListInsertArticleLink|ChangesListInsertArticleLink]] || ChangesList.php || <translate>Override or augment link to article in RC list.</translate> |
| <span style="display:none">1.120</span>1.12.0 || [[Special:MyLanguage/Manual:Hooks/ChangesListInsertArticleLink|ChangesListInsertArticleLink]] || ChangesList.php || <translate>Override or augment link to article in RC list.</translate> |
||
|- |
|- |
||
| <span style="display:none">1.280</span>1.28.0 || [[Special:MyLanguage/Manual:Hooks/ |
| <span style="display:none">1.280</span>1.28.0 || [[Special:MyLanguage/Manual:Hooks/ChangeTagsAfterUpdateTags|ChangeTagsAfterUpdateTags]] || ChangeTags.php || <translate>Called after adding or updating change tags</translate> |
||
|- |
|- |
||
| <span style="display:none">1.190</span>1.19.0 || [[Special:MyLanguage/Manual:Hooks/Collation::factory|Collation::factory]] || Collation.php ||<translate>Allows extensions to register new collation names, to be used with [[Special:MyLanguage/manual:$wgCategoryCollation|$wgCategoryCollation]]</translate> |
| <span style="display:none">1.190</span>1.19.0 || [[Special:MyLanguage/Manual:Hooks/Collation::factory|Collation::factory]] || Collation.php ||<translate>Allows extensions to register new collation names, to be used with [[Special:MyLanguage/manual:$wgCategoryCollation|$wgCategoryCollation]]</translate> |
Revision as of 23:30, 9 October 2016
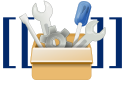
Hooks allow custom code to be executed when some defined event (such as saving a page or a user logging in) occurs.
For example, the following code snippet will trigger a call to the function MyExtensionHooks::pageContentSaveComplete
whenever the PageContentSaveComplete
hook runs, passing it function arguments specific to PageContentSaveComplete :
$wgHooks['PageContentSaveComplete'][] = 'MyExtensionHooks::pageContentSaveComplete';
MediaWiki provides many hooks like this to extend the functionality of the MediaWiki software. Assigning a function (known as an event handler) to a hook will cause that function to be called at the appropriate point in the main MediaWiki code, to perform whatever additional task(s) the developer thinks would be useful at that point. Each hook can have multiple handlers assigned to it, in which case it will call the functions in the order that they are assigned, with any modifications made by one function passed on to subsequent functions in the chain.
Assign functions to hooks at the end of LocalSettings.php or in your own extension file at the file scope (not in a $wgExtensionFunctions function or the ParserFirstCallInit hook). For extensions, if the hook function's behavior is conditioned on a setting in LocalSettings.php, the hook should be assigned and the function should terminate early if the condition was not met.
You can also create new hooks in your own extension; if you do so, add them to the Special:MyLanguage/Extension hook registry.
Background
A hook is triggered by a call to the function Hooks::run (described in file hooks.txt, and defined in GlobalFunctions.php). The first argument to Hooks::run is the name of the hook, the second is the array of arguments for that hook. It will find the event handlers to run in the array $wgHooks. It calls the PHP function call_user_func_array with arguments being the function to be called and its arguments.
See also the hook specification in core.
In this example from the doEditContent
function in WikiPage.php, doEditContent calls Hooks::run to run the PageContentSaveComplete hook, passing $hook_args
as argument:
Hooks::run( 'PageContentSaveComplete', $hook_args );
The Special:MyLanguage/core calls many hooks, but Special:MyLanguage/extensions can also call hooks.
Writing an event handler
An event handler is a function you assign to a hook, which will be run whenever the event represented by that hook occurs. It consists of:
- a function with some optional accompanying data, or
- an object with a method and some optional accompanying data.
Register the event handler by adding it to the global $wgHooks
array for a given event. Hooks can be added from any point in the execution before the hook is called, but are most commonly added in LocalSettings.php, its included files, or, for extensions, in the file extension.json. All the following are valid ways to define a hook function for the event EventName that is passed two parameters, showing the code that will be executed when EventName happens:
Format
Syntax
Resulting function call.
Static function
$wgHooks['EventName'][] = 'MyExtensionHooks::onEventName';
MyExtensionHooks::onEventName( $param1, $param2 );
Function, no data
$wgHooks['EventName'][] = 'someFunction';
someFunction( $param1, $param2 );
Function with data
$wgHooks['EventName'][] = array( 'someFunction', $someData );
someFunction( $someData, $param1, $param2 );
Function, no data
(weird syntax, but OK)
$wgHooks['EventName'][] = array( 'someFunction' );
someFunction( $param1, $param2 );
inline anonymous function
$wgHooks['EventName'][] = function( $param1, $param2 ) {
... function body
};
(the anonymous function is called with the hook's parameters)
Object only
$wgHooks['EventName'][] = $object;
$object->onEventName( $param1, $param2 );
Object with method
$wgHooks['EventName'][] = array( $object, 'someMethod' );
$object->someMethod( $param1, $param2 );
Object with method and data
$wgHooks['EventName'][] = array( $object, 'someMethod', $someData );
$object->someMethod( $someData, $param1, $param2 );
Object only
(weird syntax, but OK)
$wgHooks['EventName'][] = array( $object );
$object->onEventName( $param1, $param2 );
For extensions, the syntax is similar in the file extension.json
(corresponding to the first and second case above):
{
"Hooks": {
"EventName": [
"MyExtensionHooks::onEventName",
"someFunction"
]
}
}
When an event occurs, the function (or object method) that you registered will be called the event's parameters along with any optional data you provided at registration. Note that when an object is the hook and you didn't specify a method, the method called is 'onEventName'. For other events this would be 'onArticleSave', 'onUserLogin', etc.
The optional data is useful if you want to use the same function or object for different purposes. For example:
$wgHooks['PageContentSaveComplete'][] = array( 'ircNotify', 'TimStarling' );
$wgHooks['PageContentSaveComplete'][] = array( 'ircNotify', 'brion' );
This code would result in ircNotify being run twice when a page is saved: once for 'TimStarling', and once for 'brion'.
Event handlers can return one of three possible values:
- no return value (or null): the hook handler has operated successfully. (Before MediaWiki 1.23, returning true was required.)
- "some string": an error occurred; processing should stop and the error should be shown to the user
- false: the hook handler has done all the work necessary, or replaced normal handling. This will prevent further handlers from being run, and in some cases tells the calling function to skip normal processing.
In many cases where an error message is expected, the hook will define a variable passed as a reference for extensions to store an error message and this is preferred over returning a string that will simply be displayed as an "internal error."
The last result would be for cases where the hook function replaces the main functionality. For example, if you wanted to authenticate users to a custom system (LDAP, another PHP program, whatever), you could do:
$wgHooks['UserLogin'][] = array( 'ldapLogin', $ldapServer );
$ldap['server'] = "ldaps://ldap.company.com/";
$ldap['port'] = 636;
$ldap['base'] = ",ou=Staff,dc=company,dc=com";
function ldapLogin( $username, $password ) {
global $ldap;
$auth_user = "uid=" . $username . $ldap[ 'base' ];
wfSupressWarnings();
if ( $connect = ldap_connect( $ldap[ 'server' ], $ldap[ 'port' ] ) ){
if ( $bind = ldap_bind( $connect, $auth_user, $password ) ){
ldap_close( $connect );
return true;
} else { // if bound to ldap
echo 'Error on ldap_bind';
}
} else { // if connected to ldap
echo 'Error on ldap_connect';
}
ldap_close( $connect );
wfRestoreWarnings();
return false;
}
Returning false makes less sense for events where the action is complete, and will normally be ignored by the caller.
Documentation
Currently, hooks in MediaWiki core have to be documented both in docs/hooks.txt (in the source code repository) and here on MediaWiki.org. In some cases, one of these steps may not yet have been completed, so if a hook appears undocumented, check both.
To document a hook on-wiki, use {{MediaWikiHook }}.
Available hooks
This page contains a list of hooks that are made available by the MediaWiki software, and is known to be complete to version 1.8.2. There is a lot of detail missing for the more recent hooks in particular, as their purpose/usage has not yet been documented by the developers. If you have any further information on any of these then please add it in the appropriate place.
In the tables, the first column gives the MediaWiki version that the hook was introduced in; use the link in the second column to find out more information about the hook and how to use it.
For a complete list of hooks, use the category, which should be kept more up to date
Hooks grouped by function
Some of these hooks can be grouped into multiple functions.
- Sections: Article Management - Edit Page - Page Rendering - User Interface - Special Pages - User Management - Logging - Skinning Templates - API - Import/Export - Miscellaneous
Function
Version
Hook
Description
Article Management
1.4.0
ArticleDelete
Occurs whenever the software receives a request to delete an article
1.4.0
ArticleDeleteComplete
Occurs after the delete article request has been processed
1.9.1
ArticleUndelete
When one or more revisions of an article are restored
1.12.0
ArticleRevisionUndeleted
Occurs after an article revision is restored
1.8.0
ArticleFromTitle
Called to determine the class to handle the article rendering, based on title
1.4.0
ArticleProtect
Occurs whenever the software receives a request to protect an article
1.4.0
ArticleProtectComplete
Occurs after the protect article request has been processed
1.6.0
ArticleInsertComplete
Occurs after a new article has been created
1.11.0
RevisionInsertComplete
Called after a revision is inserted into the DB
1.4.0
ArticleSave
(Deprecated; use PageContentSave) Occurs whenever the software receives a request to save an article
1.21.0
PageContentSave
Occurs whenever the software receives a request to save an article
1.4.0
ArticleSaveComplete
(deprecated; use PageContentSaveComplete) Occurs after the save article request has been processed
1.21.0
PageContentSaveComplete
Occurs after the save article request has been processed
1.11.0
ArticleUpdateBeforeRedirect
Occurs after a page is updated (usually on save), before the user is redirected back to the page
1.5.7
ArticleEditUpdateNewTalk
Allows an extension to prevent user notification when a new message is added to their talk page.
1.14.0
ArticleEditUpdates
Called when edit updates (mainly link tracking) are made when an article has been changed.
1.6.0
ArticleEditUpdatesDeleteFromRecentchanges
Occurs before saving to the database. If returning false old entries are not deleted from the recentchangeslist.
1.8.0
RecentChange_save
Called after a "Recent Change" is committed to the DB
1.6.0
SpecialMovepageAfterMove
Called after a page is moved.
1.22.0
TitleMove
Occurs before a requested pagemove is performed
1.4.0
TitleMoveComplete
Occurs whenever a request to move an article is completed
1.12.0
AbortMove
Allows to abort moving page from one title to another
1.12.0
ArticleRollbackComplete
Occurs after an article rollback is completed
1.12.0
ArticleMergeComplete
after merging to article using Special:Mergehistory
1.6.0
ArticlePurge
Allows an extension to cancel a purge.
1.13.0
ArticleRevisionVisibilitySet
called when changing visibility of one or more revisions of an article
1.21.0
ContentHandlerDefaultModelFor
called when deciding the default content model for a given title.
1.21.0
ContentHandlerForModelID
called when a ContentHandler is requested for a given content model name, but no entry for that model exists in $wgContentHandlers.
1.23.0
Article::MissingArticleConditions
called when showing a page.
1.20.0
TitleIsAlwaysKnown
Allows overriding default behaviour for determining if a page exists.
Edit Page
1.6.0
AlternateEdit
Used to replace the entire edit page, altogether.
1.21.0
AlternateEditPreview
Allows replacement of the edit preview
1.6.0
EditFilter
Perform checks on an edit
1.12.0
EditFilterMerged
Perform checks on an edit
1.7.0
EditFormPreloadText
Called when edit page for a new article is shown. This lets you fill the text-box of a new page with initial wikitext.
1.8.3
EditPage::attemptSave
Called before an article is saved, that is before insertNewArticle() is called
1.6.0
EditPage::showEditForm:fields
Allows injection of form field into edit form.
1.6.0
EditPage::showEditForm:initial
Allows injection of HTML into edit form
1.21.0
EditPage::showStandardInputs:options
Allows injection of form fields into the editOptions area
1.13.0
EditPageBeforeConflictDiff
Allows modifying the EditPage object and output when there's an edit conflict.
1.12.0
EditPageBeforeEditButtons
Used to modify the edit buttons on the edit form
1.14.0
EditPageBeforeEditChecks
Allows modifying the edit checks below the textarea in the edit form
Page Rendering
1.27.0
AfterBuildFeedLinks
Executed after all feed links are created.
1.6.0
ArticlePageDataBefore
Executes before data is loaded for the article requested.
1.6.0
ArticlePageDataAfter
Executes after loading the data of an article from the database.
1.6.0
ArticleViewHeader
Called after an articleheader is shown.
1.6.0
PageRenderingHash
Alter the parser cache option hash key.
1.6.0
ArticleAfterFetchContent
Used to process raw wiki code after most of the other parser processing is complete.
1.22.0
GalleryGetModes
Allows extensions to add classes that can render different modes of a gallery.
1.6.0
ParserClearState
Called at the end of Parser::clearState()
1.21.0
ParserCloned
Called when the Parser object is cloned.
1.5.0
ParserBeforeStrip
Used to process the raw wiki code before any internal processing is applied.
1.5.0
ParserAfterStrip
(Deprecated) Before version 1.14.0, used to process raw wiki code after text surrounded by <nowiki>
tags have been protected but before any other wiki text has been processed. In version 1.14.0 and later, runs immediately after ParserBeforeStrip.
1.6.0
ParserBeforeInternalParse
Replaces the normal processing of stripped wiki text with custom processing. Used primarily to support alternatives (rather than additions) to the core MediaWiki markup syntax.
1.6.0
ParserGetVariableValueVarCache
Use this to change the value of the variable cache or return false to not use it.
1.6.0
ParserGetVariableValueTs
Use this to change the value of the time for the {{LOCAL...}} magic word.
1.6.0
ParserGetVariableValueSwitch
Assigns a value to a user defined variable.
1.10.0
InternalParseBeforeLinks
Used to process the expanded wiki code after <nowiki>, HTML-comments, and templates have been treated. Suitable for syntax extensions that want to customize the treatment of internal link syntax, i.e. [[....]]
.
1.13.0
LinkerMakeExternalLink
Called before the HTML for external links is returned. Used for modifying external link HTML.
1.13.0
LinkerMakeExternalImage
Called before external image HTML is returned. Used for modifying external image HTML.
1.23.0
LinkerMakeMediaLinkFile
Called before the HTML for media links is returned. Used for modifying media link HTML.
1.11.0
EditSectionLink
(Deprecated) Override the return value of Linker::editSectionLink(). Called after creating [edit] link in header in Linker::editSectionLink but before HTML is displayed.
1.11.0
EditSectionLinkForOther
(Deprecated) Override the return value of Linker::editSectionLinkForOther(). Called after creating [edit] link in header in Linker::editSectionLinkForOther but before HTML is displayed.
1.5.0
ParserBeforeTidy
Used to process the nearly-rendered html code for the page (but before any html tidying occurs).
1.5.0
ParserAfterTidy
Used to add some final processing to the fully-rendered page output.
1.24.0
ContentGetParserOutput
Customize parser output for a given content object, called by AbstractContent::getParserOutput. May be used to override the normal model-specific rendering of page content.
1.8.0
OutputPageParserOutput
Called after parse, before the HTML is added to the output.
1.6.0
OutputPageBeforeHTML
Called every time wikitext is added to the OutputPage, after it is parsed but before it is added. Called after the page has been rendered, but before the HTML is displayed.
1.4.3
CategoryPageView
Called before viewing a categorypage in CategoryPage::view
1.5.1
ArticleViewRedirect
Allows an extension to prevent the display of a "Redirected From" link on a redirect page.
1.10.0
IsFileCacheable
Allow an extension to disable file caching on pages.
1.10.1
BeforeParserFetchTemplateAndtitle
Allows an extension to specify a version of a page to get for inclusion in a template.
1.18.0
BeforeParserFetchFileAndTitle
Allows an extension to select a different version of an image to link to.
1.10.1
BeforeParserrenderImageGallery
Allows an extension to modify an image gallery before it is rendered.
1.17.0
ResourceLoaderRegisterModules
Allows registering modules with ResourceLoader
1.24.0
SidebarBeforeOutput
Directly before the sidebar is output
User Interface
1.5.4
AutoAuthenticate
Called to authenticate users on external/environmental means
1.4.0
UserLoginComplete
Occurs after a user has successfully logged in
1.18.0
BeforeWelcomeCreation
Allows an extension to change the message displayed upon a successful login.
1.4.0
UserLogout
Occurs when the software receives a request to log out
1.4.0
UserLogoutComplete
Occurs after a user has successfully logged out
1.6.0
userCan
To interrupt/advise the "user can do X to Y article" check
1.4.0
WatchArticle
Occurs whenever the software receives a request to watch an article
1.4.0
WatchArticleComplete
Occurs after the watch article request has been processed
1.4.0
UnwatchArticle
Occurs whenever the software receives a request to unwatch an article
1.4.0
UnwatchArticleComplete
Occurs after the unwatch article request has been processed
1.6.0
MarkPatrolled
Called before an edit is marked patrolled
1.6.0
MarkPatrolledComplete
Called after an edit is marked patrolled
1.4.0
EmailUser
Occurs whenever the software receives a request to send an email from one user to another
1.4.0
EmailUserComplete
Occurs after an email has been sent from one user to another
1.6.0
SpecialMovepageAfterMove
Called after a page is moved.
1.19.0
SpecialSearchCreateLink
Called when making the message to create a page or go to an existing page
1.17.0
SpecialSearchGo
1.6.0
SpecialSearchNogomatch
1.19.0
SpecialSearchPowerBox
the equivalent of SpecialSearchProfileForm for the advanced form
1.5.7
ArticleEditUpdateNewTalk
1.5.7
UserRetrieveNewTalks
1.5.7
UserClearNewTalkNotification
1.6.0
ArticlePurge
File Upload
1.6.0
UploadVerification
(Deprecated; use UploadVerifyFile) Called when a file is uploaded, to allow extra file verification to take place
1.17
UploadVerifyFile
Called when a file is uploaded, to allow extra file verification to take place (preferred)
1.6.4
UploadComplete
Called when a file upload has completed.
Special pages
1.6.0
SpecialPageGetRedirect
1.24.0
SpecialBlockModifyFormFields
Add or modify block fields of Special:Block
1.28.0
SpecialContributions::formatRow::flags
Called before rendering a Special:Contributions row.
1.13.0
SpecialListusersDefaultQuery
Called right before the end of UsersPager::getDefaultQuery()
1.13.0
SpecialListusersFormatRow
Called right before the end of UsersPager::formatRow()
1.13.0
SpecialListusersHeader
Called before closing the <fieldset> in UsersPager::getPageHeader()
1.13.0
SpecialListusersHeaderForm
Called before adding the submit button in UsersPager::getPageHeader()
1.13.0
SpecialListusersQueryInfo
Called right before the end of UsersPager::getQueryInfo()
1.6.0
SpecialPageExecuteBeforeHeader
1.6.0
SpecialPageExecuteBeforePage
1.6.0
SpecialPageExecuteAfterPage
1.6.0
SpecialVersionExtensionTypes
SpecialPage_initList
Called after the Special Page list is populated
1.9.0
UploadForm:initial
Called just before the upload form is generated
1.9.0
UploadForm:BeforeProcessing
Called just before the file data (for example description) are processed, so extensions have a chance to manipulate them.
1.14.0
UserrightsChangeableGroups
Called after the list of groups that can be manipulated via Special:UserRights is populated, but before it is returned.
User Management
1.5.0
AddNewAccount
Called after a user account is created
1.27.0
SessionMetadata
Add metadata to a session being saved
1.27.0
SessionCheckInfo
Validate session info as it's being loaded from storage
1.27.0
SecuritySensitiveOperationStatus
Affect the return value from AuthManager::securitySensitiveOperationStatus()
1.27.0
UserLoggedIn
Called after a user is logged in
1.4.0
BlockIp
Occurs whenever the software receives a request to block an IP address or user
1.4.0
BlockIpComplete
Occurs after the request to block an IP or user has been processed
1.6.0
UserRights
Called after a user's group memberships are changed
1.6.0
GetBlockedStatus
Fired after the user's getBlockStatus is set
Logging
1.6.0
LogPageActionText
1.5.0
LogPageLogHeader
1.5.0
LogPageLogName
1.5.0
LogPageValidTypes
1.26.0
LogException
Skinning / Templates
1.7.0
BeforePageDisplay
Allows last minute changes to the output page, e.g. adding of CSS or Javascript by extensions.
1.6.0
MonoBookTemplateToolboxEnd
Called by Monobook skin after toolbox links have been rendered (useful for adding more)
1.7.0
PersonalUrls
(SkinTemplate.php) Called after the list of personal URLs (links at the top in Monobook) has been populated.
1.24.0
PostLoginRedirect
(SpecialUserlogin.php) Modify the post login redirect behavior.
1.23.0
BaseTemplateAfterPortlet
(SkinTemplate.php) After rendering of portlets.
1.11.0
SkinAfterBottomScripts
(Skin.php) At the end of Skin::bottomScripts()
1.12.0
SkinSubPageSubtitle
(Skin.php) Called before the list of subpage links on top of a subpage is generated
1.5.0
SkinTemplateContentActions
Called after the default tab list is populated (list is context dependent i.e. "normal" article or "special page").
1.16.0
SkinTemplateNavigation
Called on content pages only after tabs have been added, but before variants have been added. See the other two SkinTemplateNavigation hooks for other points tabs can be modified at.
1.18.0
SkinTemplateNavigation::Universal
Called on both content and special pages after variants have been added
1.18.0
SkinTemplateNavigation::SpecialPage
Called on special pages after the special tab is added but before variants have been added
1.6.0
SkinTemplatePreventOtherActiveTabs
Called to enable/disable the inclusion of additional tabs to the skin.
1.6.0
SkinTemplateSetupPageCss
1.6.0
SkinTemplateBuildContentActionUrlsAfterSpecialPage
1.6.0
SkinTemplateBuildNavUrlsNav_urlsAfterPermalink
Called after the permalink has been entered in navigation URL array.
1.23.0
SkinTemplateGetLanguageLink
Called after building the data for a language link from which the actual html is constructed.
1.27.0
AuthChangeFormFields
Allows modification of AuthManager-based forms
1.25.0
LoginFormValidErrorMessages
Allows to add additional error messages (SpecialUserLogin.php).
1.25.0
MinervaDiscoveryTools
Allow other extensions to add or override discovery tools (SkinMinerva.php).
API
1.23.0
ApiBeforeMain
Called before ApiMain is executed
1.13.0
APIEditBeforeSave
Called right before saving an edit submitted through api.php?action=edit
1.13.0
APIQueryInfoTokens
Use this hook to add custom tokens to prop=info
1.13.0
APIQueryRevisionsTokens
Use this hook to add custom tokens to prop=revisions
1.14.0
APIQueryRecentChangesTokens
Use this hook to add custom tokens to list=recentchanges
1.14.0
APIGetAllowedParams
Use this hook to modify a module's parameters
1.14.0
APIGetParamDescription
Use this hook to modify a module's parameter descriptions
1.14.0
APIAfterExecute
Use this hook to extend core API modules
1.14.0
APIQueryAfterExecute
Use this hook to extend core API query modules
1.14.0
APIQueryGeneratorAfterExecute
Use this hook to extend core API query modules
1.23.0
GetExtendedMetadata
Allows including additional file metadata information in the imageinfo API.
1.23.0
AddNewAccountApiForm
Allows modifying the internal login form when creating an account via the API.
1.23.0
AddNewAccountApiResult
Modifies the API output when an account is created via the API.
1.25.0
ApiOpenSearchSuggest
Called when constructing the OpenSearch results. Hooks can alter or append to the array.
Import/Export
1.17.0
AfterImportPage
When a page import is completed
1.17.0
ImportHandleLogItemXMLTag
When parsing a XML tag in a log item
1.17.0
ImportHandlePageXMLTag
When parsing a XML tag in a page
1.17.0
ImportHandleRevisionXMLTag
When parsing a XML tag in a page revision
1.17.0
ImportHandleToplevelXMLTag
When parsing a top level XML tag
1.17.0
ImportHandleUploadXMLTag
When parsing a XML tag in a file upload
1.16.0
ModifyExportQuery
Modify the query used by the exporter.
1.15.0
WikiExporter::dumpStableQuery
Get the SELECT query for "stable" revisions dumps
1.16.0
XmlDumpWriterOpenPage
Called at the end of XmlDumpWriter::openPage, to allow extra metadata to be added.
1.16.0
XmlDumpWriterWriteRevision
Called at the end of a revision in an XML dump, to add extra metadata.
Miscellaneous
1.6.0
ArticleEditUpdatesDeleteFromRecentchanges
1.19.0
Collation::factory
Allows extensions to register new collation names, to be used with $wgCategoryCollation
1.18.0
GetDefaultSortkey
Allows to override what the default sortkey is, which is used to order pages in a category.
1.25.0
GetDifferenceEngine
Allows custom difference engine extensions such as Special:MyLanguage/Extension:WikEdDiff.
1.6.0
GetInternalURL
Used to modify fully-qualified URLs (useful for squid cache purging)
1.6.0
GetLocalURL
Used to modify local URLs as output into page links
1.6.0
GetFullURL
Used to modify fully-qualified URLs used in redirects/export/offsite data
1.16.0
ImgAuthBeforeStream
Executed before file is streamed to user, but only when using img_auth
1.14.0
LinkBegin
Used when generating internal and interwiki links in Linker::link()
1.22.0
LanguageLinks
Manipulate a page's language links.
1.14.0
LinkEnd
Used when generating internal and interwiki links in Linker::link(), just before the function returns a value.
1.6.0
MagicWordMagicWords
1.6.0
MagicWordwgVariableIDs
1.21.0
GetDoubleUnderscoreIDs
hook for modifying the list of magic words
1.5.7
MessagesPreLoad
Occurs when loading a message from the database
1.6.0
ParserTestParser
1.5.0
SpecialContributionsBeforeMainOutput
1.12.0
MediaWikiPerformAction
Override MediaWiki::performAction()
1.17.0
UnitTestsList
Add tests that should be run as part of the unit test suite.
1.4.0
UnknownAction
Used to add new query-string actions
1.6.0
wgQueryPages
1.8.0
DisplayOldSubtitle
1.8.0
LoadAllMessages
1.8.0
RecentChange_save
Called after a "Recent Change" is committed to the DB
1.19.0
AlternateUserMailer
Called before mail is sent so that mail could be logged (or something else) instead of using PEAR or PHP's mail().
1.24.0
UserMailerChangeReturnPath
Called to generate a VERP return address when UserMailer sends an email, with a bounce handling extension.
1.8.0
UserToggles
(Deprecated) Called before returning user preference names. Use the GetPreferences hook instead.
Alphabetical list of hooks
Version
Hook
Called From
Description
1.18.0AbortAutoAccount
SpecialUserlogin.php
Allow to cancel automated local account creation, where normally authentication against an external auth plugin would be creating a local account.
1.13.0AbortAutoblock
Block.php
Allow extension to cancel an autoblock
1.14.0AbortDiffCache
DifferenceEngine.php
Can be used to cancel the caching of a diff
1.20.0AbortEmailNotification
RecentChange.php
Can be used to cancel email notifications for an edit
1.12.0AbortMove
Title.php, SpecialMovepage.php
Can be used to cancel page movement
1.18.0ActionBeforeFormDisplay
Action.php
Before executing the HTMLForm object
1.18.0ActionModifyFormFields
Action.php
Before creating an HTMLForm object for a page action
1.5.0AddNewAccount
SpecialUserlogin.php
Called after a user account is created
1.27.0AfterBuildFeedLinks
OutputPage.php
Executed after all feed links are created.
1.20.0AfterFinalPageOutput
OutputPage.php
Nearly at the end of OutputPage::output().
1.17.0AfterImportPage
Import.php
When a page import is completed
1.17.0AfterUserMessage
User.php
After a user message has been left
1.9.1AjaxAddScript
OutputPage.php
Called in output page just before the initialisation
1.6.0AlternateEdit
EditPage.php
Occurs whenever action=edit is called
1.21.0AlternateEditPreview
EditPage.php
Allows replacement of the edit preview
1.19.0AlternateUserMailer
UserMailer.php
Called before mail is sent so that mail could be logged (or something else) instead of using PEAR or PHP's mail().
1.14.0APIAfterExecute
ApiMain.php
Use this hook to extend core API modules
1.23.0ApiBeforeMain
api.php
Called before ApiMain is executed
1.20.0ApiCheckCanExecute
ApiMain.php
Called during ApiMain::checkCanExecute()
.
1.13.0APIEditBeforeSave
ApiEditPage.php
Called right before saving an edit submitted through api.php?action=edit
1.14.0APIGetAllowedParams
ApiBase.php
Use this hook to modify a module's parameters
1.19.0APIGetDescription
ApiBase.php
Use this hook to modify a module's descriptions
1.14.0APIGetParamDescription
ApiBase.php
Use this hook to modify a module's parameter descriptions
1.20.0APIGetResultProperties
ApiBase.php
Use this hook to modify the properties in a module's result.
1.20.0ApiMain::onException
ApiMain.php
Called by ApiMain::executeActionWithErrorHandling()
when an exception is thrown during API action execution.
1.25.0ApiOpenSearchSuggest
ApiOpenSearch.php
Called when constructing the OpenSearch results.
1.20.0ApiTokensGetTokenTypes
ApiTokens.php
Use this hook to extend action=tokens with new token types
1.14.0APIQueryAfterExecute
ApiQuery.php
Use this hook to extend core API query modules
1.14.0APIQueryGeneratorAfterExecute
ApiQuery.php
Use this hook to extend core API query modules
1.13.0APIQueryInfoTokens
ApiQueryInfo.php
Use this hook to add custom tokens to prop=info.
1.14.0APIQueryRecentChangesTokens
ApiQueryRecentChanges.php
Use this hook to add custom tokens to list=recentchanges.
1.13.0APIQueryRevisionsTokens
ApiQueryRevisions.php
Use this hook to add custom tokens to prop=revisions.
1.15.0APIQueryUsersTokens
ApiQueryUsers.php
Use this hook to add custom tokens to list=users.
1.17.0ApiRsdServiceApis
ApiRsd.php
Add or remove APIs from the RSD services list.
1.23.0Article::MissingArticleConditions
Article.php
Called when an article is shown.
1.6.0ArticleAfterFetchContent
Article.php
Used to process raw wiki code after most of the other parser processing is complete.
1.16.0ArticleConfirmDelete
Article.php
Occurs before writing the confirmation form for article deletion.
1.17.0ArticleContentOnDiff
DifferenceEngine.php
Before showing the article content below a diff.
1.4.0ArticleDelete
Article.php
Occurs whenever the software receives a request to delete an article
1.4.0ArticleDeleteComplete
Article.php
Occurs after the delete article request has been processed
1.5.7ArticleEditUpdateNewTalk
Article.php
Allows an extension to prevent user notification when a new message is added to their talk page.
1.14.0ArticleEditUpdates
Article.php
Called when edit updates (mainly link tracking) are made when an article has been changed.
1.6.0ArticleEditUpdatesDeleteFromRecentchanges
Article.php
Occurs before saving to the database. If returning false old entries are not deleted from the recentchangeslist.
1.8.0ArticleFromTitle
Wiki.php
Called to determine the class to handle the article rendering, based on title.
1.6.0ArticleInsertComplete
Article.php
Called after an article is created
1.12.0ArticleMergeComplete
SpecialMergeHistory.php
after merging to article using Special:Mergehistory
1.6.0ArticlePageDataAfter
Article.php
after loading data of an article from the database
1.6.0ArticlePageDataBefore
Article.php
before loading data of an article from the database
1.18.0ArticlePrepareTextForEdit
Article.php
Called when preparing text to be saved.
1.4.0ArticleProtect
Article.php
Occurs whenever the software receives a request to protect an article
1.4.0ArticleProtectComplete
Article.php
Occurs after the protect article request has been processed
1.6.0ArticlePurge
Article.php
Allows an extension to cancel a purge.
1.13.0ArticleRevisionVisiblitySet
SpecialRevisiondelete.php
called when changing visibility of one or more revisions of an article
1.12.0ArticleRevisionUndeleted
SpecialUndelete.php
Occurs after an article revision is restored
1.12.0ArticleRollbackComplete
Article.php
Occurs after an article rollback is completed
1.4.0ArticleSave
Article.php
Occurs whenever the software receives a request to save an article
1.4.0ArticleSaveComplete
Article.php
Occurs after the save article request has been processed
1.9.1ArticleUndelete
SpecialUndelete.php
When one or more revisions of an article are restored
1.11.0ArticleUpdateBeforeRedirect
Article.php
Occurs after a page is updated (usually on save), before the user is redirected back to the page
1.19.0ArticleViewCustom
Article.php
Allows to output the text of the article in a different format than wikitext.
1.18.0ArticleViewFooter
Article.php
After showing the footer section of an ordinary page view.
1.6.0ArticleViewHeader
Article.php
Occurs when header is shown
1.5.1ArticleViewRedirect
Article.php
Allows an extension to prevent the display of a "Redirected From" link on a redirect page.
1.27.0AuthChangeFormFields
AuthManagerSpecialPage.php
Allows modification of AuthManager-based forms
1.13.0AuthPluginAutoCreate
SpecialUserlogin.php
Called when creating a local account for an user logged in from an external authentication method
1.9.1AuthPluginSetup
Setup.php
Update or replace authentication plugin object ($wgAuth)
1.5.4AutoAuthenticate
StubObject.php
Called to authenticate users on external/environmental means.
1.12.0AutopromoteCondition
Autopromote.php
check autopromote condition for user.
1.19.0BacklinkCacheGetConditions
BacklinkCache.php
Allows to set conditions for query when links to certain title.
1.19.0BacklinkCacheGetPrefix
BacklinkCache.php
Allows to set prefix for a spefific link table.
1.7.0BadImage
ImageFunctions.php
Before bad image list is evaluated
1.23.0BaseTemplateAfterPortlet
SkinTemplate.php
Called after rendering of portlets.
1.18.0BaseTemplateToolbox
SkinTemplate.php
Called by BaseTemplate when building the toolbox array
and returning it for the skin to output.
1.10.1BeforeGalleryFindFile
ImageGallery.php
Allows extensions to specify a specific version of an image to display in a gallery.
1.19.0BeforeDisplayNoArticleText
Article.php
Before displaying noarticletext or noarticletext-nopermission messages.
1.16.0BeforeInitialize
Wiki.php
Occurs before anything is initialized in MediaWiki::performRequestForTitle().
1.7.0BeforePageDisplay
OutputPage.php
SkinTemplate.php (< 1.12.0)
Allows last minute changes to the output page, e.g. adding of CSS or Javascript by extensions.
1.19.0BeforePageRedirect
OutputPage.php
Called prior to sending an HTTP redirect.
1.18.0BeforeParserFetchFileAndTitle
Parser.php
Before an image is rendered by Parser
1.10.1BeforeParserFetchTemplateAndtitle
Parser.php
Before a template is fetched by Parser
1.10.1BeforeParserrenderImageGallery
Parser.php
Before an image gallery is rendered by Parser
1.12.0BeforeWatchlist
SpecialWatchlist.php
Override watchlist display or add extra SQL clauses.
1.18.0BeforeWelcomeCreation
SpecialUserlogin.php
Allows an extension to change the message displayed upon a successful login.
1.19.0BitmapHandlerCheckImageArea
Bitmap.php
Called by BitmapHandler::normaliseParams()
, after all normalizations have been performed, except for the $wgMaxImageArea check.
1.18.0BitmapHandlerTransform
Bitmap.php
Before a file is transformed, gives extension the possibility to transform it themselves.
1.4.0BlockIp
SpecialBlockip.php
Occurs whenever the software receives a request to block an IP address or user
1.4.0BlockIpComplete
SpecialBlockip.php
Occurs after the request to block an IP or user has been processed
1.9.1BookInformation
SpecialBooksources.php
Before information output on Special:Booksources
1.13.0BrokenLink
Linker.php
Before the HTML is created for a broken (i.e. red) link
1.17.0CanonicalNamespaces
Namespace.php
For extensions adding their own namespaces or altering the defaults.
1.4.3CategoryPageView
CategoryPage.php
Called before viewing a categorypage in CategoryPage::view
1.20.0ChangePasswordForm
SpecialChangePassword.php
For extensions that need to add a field to the ChangePassword form via the Preferences form
1.12.0ChangesListInsertArticleLink
ChangesList.php
Override or augment link to article in RC list.
1.28.0ChangeTagsAfterUpdateTags
ChangeTags.php
Called after adding or updating change tags
1.19.0Collation::factory
Collation.php
Allows extensions to register new collation names, to be used with $wgCategoryCollation
1.16.0ConfirmEmailComplete
User.php
Called after a user's email has been confirmed successfully.
1.24.0ContentGetParserOutput
AbstractContent.php
Customize parser output for a given content object, called by AbstractContent::getParserOutput. May be used to override the normal model-specific rendering of page content.
1.21.0ContentHandlerDefaultModelFor
ContentHandler.php
Called when deciding the default content model for a given title.
1.21.0ContentHandlerForModelID
ContentHandler.php
Allows to provide custom content handlers for content models.
1.13.0ContribsPager::getQueryInfo
SpecialContributions.php
Before the contributions query is about to run
1.20.0ContribsPager::reallyDoQuery
SpecialContributions.php
Called before really executing the query for My Contributions
1.13.0ContributionsLineEnding
SpecialContributions.php
Called before a contributions HTML line is finished
1.11.0ContributionsToolLinks
SpecialContributions.php
Change tool links above Special:Contributions
1.9.1CustomEditor
Wiki.php
When invoking the page editor. Return true to allow the normal editor to be used, or false if implementing a custom editor, e.g. for a special namespace, etc.
1.16.0DatabaseOraclePostInit
DatabaseOracle.php
Called after initialising an Oracle database connection
1.7.0DiffViewHeader
DifferenceEngine.php
Called before diff display
1.8.0DisplayOldSubtitle
Article.php
Allows extensions to modify the displaying of links to other revisions when browsing through revisions.
1.14.0DoEditSectionLink
Linker.php
Override the HTML generated for section edit links.
1.6.0EditFilter
EditPage.php
Perform checks on an edit
1.12.0EditFilterMerged
EditPage.php
Perform checks on an edit
1.7.0EditFormPreloadText
EditPage.php
Called when edit page for a new article is shown. This lets you fill the text-box of a new page with initial wikitext.
1.16.0EditFormInitialText
EditPage.php
Called when edit page for a existing article is shown. This lets you modify the content.
1.8.3EditPage::attemptSave
EditPage.php
Called before an article is saved, that is before insertNewArticle() is called
1.16.0EditPage::importFormData
EditPage.php
Allow extensions to read additional data posted in the form
1.6.0EditPage::showEditForm:fields
EditPage.php
Allows injection of form field into edit form.
1.6.0EditPage::showEditForm:initial
EditPage.php
before showing the edit form
1.21.0EditPage::showStandardInputs:options
EditPage.php
Allows injection of form fields into the editOptions area
1.13.0EditPageBeforeConflictDiff
EditPage.php
Allows modifying the EditPage object and output when there's an edit conflict.
1.12.0EditPageBeforeEditButtons
EditPage.php
Used to modify the edit buttons on the edit form
1.14.0EditPageBeforeEditChecks
EditPage.php
Allows modifying the edit checks below the textarea in the edit form
1.16.0EditPageBeforeEditToolbar
EditPage.php
Allows modifying the edit toolbar above the textarea
1.16.0EditPageCopyrightWarning
EditPage.php
Allow for site and per-namespace customization of contribution/copyright notice.
1.16.0 EditPageGetDiffText
EditPage.php
Allow modifying the wikitext that will be used in "Show changes".
1.16.0EditPageGetPreviewText
EditPage.php
Allow modifying the wikitext that will be previewed.
1.16.0EditPageNoSuchSection
EditPage.php
When a section edit request is given for an non-existent section.
1.16.0EditPageTosSummary
EditPage.php
Give a chance for site and per-namespace customizations of terms of service summary link.
1.11.0EditSectionLink
EditPage.php
Override the return value of Linker::editSectionLink()
1.11.0EditSectionLinkForOther
EditPage.php
Override the return value of Linker::editSectionLink()
1.7.0EmailConfirmed
User.php
When checking that the user's email address is "confirmed"
1.4.0EmailUser
SpecialEmailuser.php
Occurs whenever the software receives a request to send an email from one user to another
1.17.0EmailUserCC
SpecialEmailuser.php
Occurs before sending the copy of the email to the author
1.4.0EmailUserComplete
SpecialEmailuser.php
Occurs after an email has been sent from one user to another
1.17.0EmailUserForm
SpecialEmailuser.php
Occurs after building the email user form object
1.16.0EmailUserPermissionsErrors
SpecialEmailuser.php
Retrieves permissions errors for emailing a user
1.19.0ExemptFromAccountCreationThrottle
SpecialUserlogin.php
To add an exemption from the account creation throttle
1.18.0ExtensionTypes
SpecialVersion.php
Called when generating the extensions credits, use this to change the tables headers
1.19.0ExtractThumbParameters
thumb.php
Called when extracting thumbnail parameters from a thumbnail file name.
1.7.0FetchChangesList
ChangesList.php
Allows extension to modify a recent changes list for a user.
1.13.0FileDeleteComplete
FileDeleteForm.php
When a file is deleted
1.20.0FileTransformed
File.php
When a file is transformed and moved into storage
1.11.0FileUpload
filerepo/LocalFile.php
When a file upload occurs
1.13.0FileUndeleteComplete
SpecialUndelete.php
When a file is undeleted
1.20.0FormatAutocomments
Linker.php
When an autocomment is formatted by the Linker
1.17.0FormatUserMessage
User.php
Hook to format a message if you want to override the internal formatter.
1.22.0GalleryGetModes
ImageGalleryBase.php
Allows extensions to add classes that can render different modes of a gallery.
1.13.0GetAutoPromoteGroups
Autopromote.php
When determining which autopromote groups a user is entitled to be in.
1.6.0GetBlockedStatus
User.php
Fired after the user's getBlockStatus is set.
1.13.0GetCacheVaryCookies
OutputPage.php
get cookies that should vary cache options
1.18.0GetCanonicalURL
Title.php
Allows to modify fully-qualified URLs used for IRC and e-mail notifications.
1.18.0GetDefaultSortkey
Title.php
Allows to override what the default sortkey is.
1.25.0GetDifferenceEngine
ContentHandler.php
Allows custom difference engine extensions such as ESpecial:MyLanguage/xtension:WikEdDiff.
1.23.0GetExtendedMetadata
FormatMetadata.php
Allows including additional file metadata information in the imageinfo API.
1.6.0GetFullURL
Title.php
Used to modify fully-qualified URLs used in redirects/export/offsite data
1.6.0GetInternalURL
Title.php
Used to modify fully-qualified URLs (useful for squid cache purging)
1.17.0GetIP
ProxyTools.php
Modify the ip of the current user (called only once).
1.12.0GetLinkColours
LinkHolderArray.php
modify the CSS class of an array of page links
1.6.0GetLocalURL
Title.php
Used to modify local URLs as output into page links
1.19.0GetLocalURL::Article
Title.php
Allows to modify local URLs specifically pointing to article paths without any fancy queries or variants.
1.19.0GetLocalURL::Internal
Title.php
Allows to modify local URLs to internal pages.
1.18.0GetMetadataVersion
Generic.php
Allows to modify the image metadata version currently in use.
1.15.0GetPreferences
Preferences.php
Modify user preferences
1.12.0getUserPermissionsErrors
Title.php
Add a permissions error when permissions errors are checked for.
1.12.0getUserPermissionsErrorsExpensive
Title.php
Absolutely the same, but is called only if expensive checks are enabled.
1.20.0GitViewers
GitInfo.php
Called when generating the list of git viewers for Special:Version, use this to change the list.
1.14.0HTMLCacheUpdate::doUpdate
HTMLCacheUpdate.php
After cache invalidation updates are inserted into the job queue
1.13.0ImageBeforeProduceHTML
Linker.php
Called before producing the HTML created by a wiki image insertion.
1.11.0ImageOpenShowImageInlineBefore
ImagePage.php
Call potential extension just before showing the image on an image page.
1.16.0ImagePageAfterImageLinks
ImagePage.php
Called after the image links section on an image page is built.
1.13.0ImagePageFileHistoryLine
ImagePage.php
Called when a file history line is contructed.
1.13.0ImagePageFindFile
ImagePage.php
Called when fetching the file associed with an image page.
1.16.0ImagePageShowTOC
ImagePage.php
Called when the file toc on an image page is generated.
1.17.0ImportHandleLogItemXMLTag
Import.php
When parsing a XML tag in a log item
1.17.0ImportHandlePageXMLTag
Import.php
When parsing a XML tag in a page
1.17.0ImportHandleRevisionXMLTag
Import.php
When parsing a XML tag in a page revision
1.17.0ImportHandleToplevelXMLTag
Import.php
When parsing a top level XML tag
1.17.0ImportHandleUploadXMLTag
Import.php
When parsing a XML tag in a file upload
1.20.0InfoAction
InfoAction.php
When building information to display on the action=info page
1.11.0InitPreferencesForm
SpecialPreferences.php
Called at the end of PreferencesForm's constructor
1.16.0ImgAuthBeforeStream
img_auth.php
Executed before file is streamed to user, but only when using img_auth
1.13.0InitializeArticleMaybeRedirect
Wiki.php
Called when checking if title is a redirect
1.10.0InternalParseBeforeLinks
Parser.php
Used to process the expanded wiki code after <nowiki>, HTML-comments, and templates have been treated. Suitable for syntax extensions that want to support templates and comments.
1.16.0InvalidateEmailComplete
User.php
Called after a user's email has been invalidated successfully.
1.18.0IRCLineURL
RecentChange.php
When constructing the URL to use in an IRC notification.
1.10.0IsFileCacheable
Article.php
Allow an extension to disable file caching on pages.
1.9.0IsTrustedProxy
ProxyTools.php
Allows an extension to set an IP as trusted or not.
1.12.0isValidEmailAddr
User.php
Override the result of User::isValidEmailAddr()
1.11.0isValidPassword
User.php
Override the result of User::isValidPassword()
1.19.0Language::getMessagesFileName
languages/Language.php
Use to change the path of a localisation file.
1.19.0LanguageGetNamespaces
languages/Language.php
Provide custom ordering for namespaces or
remove namespaces.
1.9.0LanguageGetSpecialPageAliases
languages/Language.php
Use to define aliases of special pages names depending of the language
1.18.0LanguageGetTranslatedLanguageNames
languages/Language.php
Provide translated language names.
1.22.0LanguageLinks
api/ApiParse.php, skin/OutputPage.php
Manipulate a page's language links.
1.14.0LinkBegin
Linker.php
Used when generating internal and interwiki links in Linker::link()
1.14.0LinkEnd
Linker.php
Used when generating internal and interwiki links in Linker::link(), just before the function returns a value.
1.13.0LinkerMakeExternalLink
Linker.php
At the end of Linker::makeExternalLink() just before the return
1.13.0LinkerMakeExternalImage
Linker.php
At the end of Linker::makeExternalImage() just before the return
1.12.0LinksUpdate
LinksUpdate.php
At the beginning of LinksUpdate::doUpdate() just before the actual update.
1.12.0LinksUpdateComplete
LinksUpdate.php
At the end of LinksUpdate::doUpdate() when updating has completed.
1.11.0LinksUpdateConstructed
LinksUpdate.php
At the end of LinksUpdate() is construction.
1.15.0ListDefinedTags
ChangeTags.php
When trying to find all defined tags.
1.8.0LoadAllMessages
MessageCache.php
called by MessageCache::loadAllMessages() to load extensions messages
1.10.1LoadExtensionSchemaUpdates
maintenance/updaters.inc
Fired when MediaWiki is updated to allow extensions to update the database.
1.13.0LocalFile::getHistory
LocalFile.php
Called before file history query performed
1.19.0LocalFilePurgeThumbnails
LocalFile.php
Called before thumbnails for a local file a purged
1.26.0LogException
MWExceptionHandler.php
Called before an exception (or PHP error) is logged
1.19.0LogEventsListShowLogExtract
LogEventsList.php
Called before the result of LogEventsList::showLogExtract()
is added to OutputPage.
1.10.0LoginAuthenticateAudit
SpecialUserlogin.php
A login attempt for a valid user account either succeeded or failed. No return data is accepted; this hook is for auditing only.
1.12.0LogLine
SpecialLog.php
Processes a single log entry on Special:Log
1.6.0LogPageActionText
Setup.php
Strings used by wfMsg as a header. DEPRECATED: Use $wgLogActions
1.5.0LogPageLogHeader
Setup.php
Strings used by wfMsg as a header. DEPRECATED: Use $wgLogHeaders
1.5.0LogPageLogName
Setup.php
Name of the logging page(s). DEPRECATED: Use $wgLogNames
1.5.0LogPageValidTypes
Setup.php
Action being logged. DEPRECATED: Use $wgLogTypes
1.6.0MagicWordwgVariableIDs
MagicWord.php
Allows extensions to add Magic Word IDs.
1.18.0MaintenanceRefreshLinksInit
maintenance/refreshLinks.php
Before executing the refreshLinks.php maintenance script.
1.14.0MakeGlobalVariablesScript
Skin.php
called right before Skin::makeVariablesScript() is executed
1.6.0MarkPatrolled
Article.php
Called before an edit is marked patrolled
1.6.0MarkPatrolledComplete
Article.php
Called after an edit is marked patrolled
1.7.0MathAfterTexvc
Math.php
After texvc is executed when rendering mathematics DEPRECATED: Use MathFormulaPostRender
1.7.0MathFormulaPostRender
Math.php
After math is rendered, before it's printed to the screen
1.12.0MediaWikiPerformAction
Wiki.php
Override MediaWiki::performAction().
1.15.0MessageCacheReplace
MessageCache.php
When a message page is changed. Useful for updating caches.
1.16.0MessageNotInMwNs
MessageCache.php
When trying to get a message that isn't found in the MediaWiki namespace
1.5.7MessagesPreLoad
MessageCache.php
Occurs when loading a message from the database
1.16.0ModifyExportQuery
Export.php
Modify the query used by the exporter.
1.6.0MonoBookTemplateToolboxEnd
skins/Monobook.php
Called by Monobook skin after toolbox links have been rendered (useful for adding more)
1.20.0NamespaceIsMovable
Namespace.php
Called when determining if it is possible to pages in a namespace.
1.15.0NewDifferenceEngine
diff/DifferenceEngine.php
Called when a new DifferenceEngine object is made.
1.13.0NewRevisionFromEditComplete
(Various files)
Called when a revision was inserted due to an edit
1.13.0NormalizeMessageKey
MessageFunctions.php
Called before the software gets the text of a message
1.14.0OldChangesListRecentChangesLine
ChangesList.php
Customize entire Recent Changes line
1.13.0OpenSearchUrls
opensearch_desc.php
Called when constructing the OpenSearch description XML.
1.16.0OtherBlockLogLink
SpecialBlockip.php
Get links to the block log from extensions which blocks users and/or IP addresses too
1.6.0OutputPageBeforeHTML
OutputPage.php
Called after the page has been rendered, but before the HTML is displayed.
1.17.0OutputPageBodyAttributes
OutputPage.php
Called when OutputPage::headElement() is creating the body tag.
1.14.0OutputPageCheckLastModified
OutputPage.php
When checking if the page has been modified since the last visit
1.13.0OutputPageMakeCategoryLinks
OutputPage.php
Called when links are about to be generated for the page's categories.
1.8.0OutputPageParserOutput
OutputPage.php
after adding a parserOutput to $wgOut
1.19.0PageContentLanguage
Title.php
Allows changing the page content language and the direction depending on that language.
1.10.0PageHistoryBeforeList
PageHistory.php
When a history page list is about to be constructed.
1.10.0PageHistoryLineEnding
PageHistory.php
Right before the end >li> is added to a history line
1.13.0PageHistoryPager::getQueryInfo
PageHistory.php
When a history pager query parameter set is constructed
1.6.0PageRenderingHash
User.php
Alter the parser cache option hash key
1.20.0ParserAfterParse
Parser.php
Called from Parser::parse() just after the call to Parser::internalParse() returns
1.5.0ParserAfterStrip
Parser.php
Same as ParserBeforeStrip
1.5.0ParserAfterTidy
Parser.php
Used to add some final processing to the fully-rendered page output
1.6.0ParserBeforeInternalParse
Parser.php
called at the beginning of Parser::internalParse()
1.5.0ParserBeforeStrip
Parser.php
Used to process the raw wiki code before any internal processing is applied
1.5.0ParserBeforeTidy
Parser.php
Used to process the nearly-rendered html code for the page (but before any html tidying occurs)
1.6.0ParserClearState
Parser.php
called at the end of Parser::clearState()
1.21.0ParserCloned
Parser.php
called when the Parser object is cloned
1.12.0ParserFirstCallInit
Parser.php
called when the parser initialises for the first time
1.6.0ParserGetVariableValueSwitch
Parser.php
called when the parser needs the value of a custom magic word
1.6.0ParserGetVariableValueTs
Parser.php
use this to change the value of the time for the {{LOCAL...}} magic word
1.6.0ParserGetVariableValueVarCache
Parser.php
use this to change the value of the variable cache or return false to not use it
1.12.0ParserLimitReport
Parser.php
called at the end of Parser::parse() when the parser will include comments about size of the text parsed
1.12.0ParserMakeImageParams
Parser.php
called at the end of Parser::makeImage(). Alter the parameters used to generate an image.
1.19.0ParserSectionCreate
Parser.php
Called each time the parser creates a document section from wikitext.
1.20.0ParserTestGlobals
parserTest.inc, NewParserTest.php
Allows to define globals for parser tests.
1.6.0ParserTestParser
parserTest.inc, NewParserTest.php
called when creating a new instance of Parser in maintenance/parserTests.inc
1.10.0ParserTestTables
maintenance/parserTests.inc
Alter the list of tables to duplicate when parser tests are run.
Use when page save hooks require the presence of custom tables to ensure that tests continue to run properly.
1.18.0PerformRetroactiveAutoblock
Block.php
Called before a retroactive autoblock is applied to a user.
1.6.0PersonalUrls
SkinTemplate.php
Alter the user-specific navigation links (e.g. "my page, my talk page, my contributions" etc).
1.9.0PingLimiter
User.php
Allows extensions to override the results of User::pingLimiter()
1.19.0PlaceNewSection
WikiPage.php
Allows extensions to control where new sections are added.
1.24.0PostLoginRedirect
SpecialUserlogin.php
Modify the post login redirect behavior.
1.20.0PreferencesGetLegend
Preferences.php
Override the text used for the <legend> of a preferences section.
1.9.0PreferencesUserInformationPanel
SpecialPreferences.php
Add HTML bits to user information list in preferences form
1.12.0PrefixSearchBackend
PrefixSearch.php
Override the title prefix search used for OpenSearch and AJAX search suggestions.
1.11.0PrefsEmailAudit
SpecialPreferences.php
called when user changes his email address
1.11.0PrefsPasswordAudit
SpecialPreferences.php
called when user changes his password
1.16.0ProtectionForm::buildForm
ProtectionForm.php
Called after all protection type fieldsets are made in the form
1.16.0ProtectionForm::save
ProtectionForm.php
Called when a protection form is submitted
1.16.0ProtectionForm::showLogExtract
ProtectionForm.php
Called after the protection log extract is shown
1.10.0RawPageViewBeforeOutput
RawPage.php
Right before the text is blown out in action=raw
1.8.0RecentChange_save
RecentChange.php
Called after a "Recent Change" is commited to the DB
1.20.0RedirectSpecialArticleRedirectParams
SpecialPage.php
Lets you alter the set of parameter names such as "oldid" that are preserved when using.
1.11.0RenderPreferencesForm
SpecialPreferences.php
Called at the end of PreferencesForm::mainPrefsForm
1.19.0RequestContextCreateSkin
RequestContext.php
Called when creating a skin instance.
1.11.0ResetPreferences
SpecialPreferences.php
Called at the end of PreferencesForm::resetPrefs
1.17.0ResourceLoaderGetConfigVars
ResourceLoaderStartUpModule.php
Called right before ResourceLoaderStartUpModule::getConfig()
returns.
1.18.0ResourceLoaderGetStartupModules
ResourceLoaderStartUpModule.php
Run once the startup module is being generated.
1.17.0ResourceLoaderRegisterModules
ResourceLoader.php
Allows registering of modules with ResourceLoader.
1.19.0ResourceLoaderTestModules
ResourceLoader.php
Add new javascript testing modules. This is called after the addition of MediaWiki core test suites.
1.11.0RevisionInsertComplete
Revision.php
Called after a revision is inserted into the DB
1.11.0SavePreferences
SpecialPreferences.php
Called at the end of PreferencesForm::savePreferences; returning false prevents the preferences from being saved.
1.16.0SearchableNamespaces
SearchEngine.php
An option to modify which namespaces are searchable.
1.28.0SearchDataForIndex
SearchEngine.php
Allows to provide custom content fields when indexing a document.
1.16.0SearchEngineReplacePrefixesComplete
SearchEngine.php
Run after SearchEngine::replacePrefixes().
1.12.0SearchGetNearMatch
SearchEngine.php
An extra chance for exact-title-matches in "go" searches.
1.16.0SearchGetNearMatchBefore
SearchEngine.php
Perform exact-title-matches in "go" searches before the normal operations.
1.16.0SearchGetNearMatchComplete
SearchEngine.php
A chance to modify exact-title-matches in "go" searches.
1.28.0SearchIndexFields
SearchEngine.php
Add fields to search index mapping.
1.20.0SearchResultInitFromTitle
SearchEngine.php
Set the revision used when displaying a page in search results.
1.10.0SearchUpdate
SearchUpdate.php
Prior to search update completion
1.27.0SecuritySensitiveOperationStatus
AuthManager.php
Affect the return value from AuthManager::securitySensitiveOperationStatus()
1.27.0SessionCheckInfo
SessionManager.php
Validate session info as it's being loaded from storage
1.27.0SessionMetadata
SessionBackend.php
Add metadata to a session being saved
1.14.0SetupAfterCache
Setup.php
Called in Setup.php, after cache objects are set
1.17.0SetupUserMessageArticle
User.php
Called in User::leaveUserMessage() before anything has been posted to the article.
1.16.0ShowMissingArticle
Article.php
Called when generating the output for a non-existent page
1.11.0ShowRawCssJs
Article.php
Customise the output of raw CSS and JavaScript in page views
1.16.0ShowSearchHitTitle
SpecialSearch.php
Customise display of search hit title/link.
1.7.0SiteNoticeAfter
GlobalFunctions.php
After site notice is determined
1.7.0SiteNoticeBefore
GlobalFunctions.php
Before site notice is determined
1.11.0SkinAfterBottomScripts
Skin.php
At the end of Skin::bottomScripts()
1.14.0SkinAfterContent
Skin.php
Allows extensions to add text after the page content and article metadata.
1.14.0SkinBuildSidebar
Skin.php
At the end of Skin::buildSidebar()
1.24.0SidebarBeforeOutput
Skin.php
Directly before the Sidebar is output
1.16.0SkinCopyrightFooter
Skin.php
Allow for site and per-namespace customization of copyright notice.
???
SkinEditSectionLinks
Skin.php
Modify the section edit links. Called when section headers are created.
1.17.0SkinGetPoweredBy
Skin.php
Called when generating the code used to display the "Powered by MediaWiki" icon.
1.12.0SkinSubPageSubtitle
Skin.php
Called before the list of subpage links on top of a subpage is generated
1.6.0SkinTemplateBuildContentActionUrlsAfterSpecialPage
SkinTemplate.php
after the single tab when showing a special page
1.6.0SkinTemplateBuildNavUrlsNav_urlsAfterPermalink
SkinTemplate.php
after creating the "permanent link" tab
1.5.0SkinTemplateContentActions
SkinTemplate.php
Alter the "content action" links in SkinTemplates
1.23.0SkinTemplateGetLanguageLink
SkinTemplate.php
Called after building the data for a language link from which the actual html is constructed.
1.16.0SkinTemplateNavigation
SkinTemplate.php
Alter the structured navigation links in SkinTemplate
1.10.0SkinTemplateOutputPageBeforeExec
SkinTemplate.php
Before SkinTemplate::outputPage() starts page output
1.6.0SkinTemplatePreventOtherActiveTabs
SkinTemplate.php
use this to prevent showing active tabs
1.6.0SkinTemplateSetupPageCss
SkinTemplate.php
use this to provide per-page CSS
1.18.0SkinTemplateNavigation::SpecialPage
SkinTemplate.php
Called on special pages after the special tab is added but before variants have been added
1.12.0SkinTemplateTabAction
SkinTemplate.php
Override SkinTemplate::tabAction().
1.6.0SkinTemplateTabs
SkinTemplate.php
called when finished to build the actions tabs
1.13.0SkinTemplateToolboxEnd
skins/Monobook.php, skins/Modern.php
Called by SkinTemplate skins after toolbox links have been rendered (useful for adding more)
1.18.0SkinTemplateNavigation::Universal
SkinTemplate.php
Called on both content and special pages after variants have been added
1.15.0SoftwareInfo
SpecialVersion.php
Called by Special:Version for returning information about the software
1.24.0SpecialBlockModifyFormFields
SpecialContributions.php
Add or modify block fields of Special:Block
1.5.0SpecialContributionsBeforeMainOutput
SpecialBlock.php
Before the form on Special:Contributions
1.28.0SpecialContributions::formatRow::flags
ContribsPager.php
Called before rendering a Special:Contributions row.
1.13.0SpecialListusersDefaultQuery
SpecialListusers.php
Called right before the end of UsersPager::getDefaultQuery()
1.13.0SpecialListusersFormatRow
SpecialListusers.php
Called right before the end of UsersPager::formatRow()
1.13.0SpecialListusersHeader
SpecialListusers.php
Called before closing the <fieldset> in UsersPager::getPageHeader()
1.13.0SpecialListusersHeaderForm
SpecialListusers.php
Called before adding the submit button in UsersPager::getPageHeader()
1.13.0SpecialListusersQueryInfo
SpecialListusers.php
Called right before the end of UsersPager::getQueryInfo()
1.6.0SpecialMovepageAfterMove
SpecialMovepage.php
Called after a page is moved.
1.18.0SpecialNewPagesFilters
SpecialNewpages.php
Called after building form options at NewPages.
1.7.0SpecialPage_initList
SpecialPage.php
Called when the list of Special Pages is populated. Hook gets a chance to change the list to hide/show pages
1.20.0SpecialPageAfterExecute
SpecialPage.php
Called after SpecialPage::execute().
1.20.0SpecialPageBeforeExecute
SpecialPage.php
Called before SpecialPage::execute().
1.6.0SpecialPageExecuteAfterPage
SpecialPage.php
called after executing a special page
1.6.0SpecialPageExecuteBeforeHeader
SpecialPage.php
called before setting the header text of the special page
1.6.0SpecialPageExecuteBeforePage
SpecialPage.php
called after setting the special page header text but before the main execution
1.18.0SpecialPasswordResetOnSubmit
SpecialPasswordReset.php
Called when executing a form submission on Special:PasswordReset.
1.16.0SpecialRandomGetRandomTitle
SpecialRandompage.php
Modify the selection criteria for Special:Random
1.18.0SpecialRecentChangesFilters
SpecialRecentchanges.php
Called after building form options at RecentChanges
1.13.0SpecialRecentChangesPanel
SpecialRecentchanges.php
called when building form options in SpecialRecentChanges
1.13.0SpecialRecentChangesQuery
SpecialRecentchanges.php
called when building sql query for SpecialRecentChanges
1.19.0SpecialSearchCreateLink
SpecialSearch.php
Called when making the message to create a page or go to the existing page.
1.6.0SpecialSearchNogomatch
SpecialSearch.php
called when user clicked the "Go" button but the target doesn't exist
1.13.0SpecialSearchNoResults
SpecialSearch.php
called before search result display when there are no matches
1.19.0SpecialSearchPowerBox
SpecialSearch.php
The equivalent of SpecialSearchProfileForm for the advanced form, a.k.a. power search box
1.18.0SpecialSearchProfileForm
SpecialSearch.php
allows modification of search profile forms
1.16.0SpecialSearchProfiles
SpecialSearch.php
allows modification of search profiles
1.13.0SpecialSearchResults
SpecialSearch.php
called before search result display when there are matches
1.26.0SpecialSearchResultsAppend
SpecialSearch.php
Called after the list of search results HTML has been output.
1.21.0SpecialSearchResultsPrepend
SpecialSearch.php
Called immediately before returning HTML on the search results page. Useful for including an external search provider. To disable the output of MediaWiki search output, return false.
1.18.0SpecialSearchSetupEngine
SpecialSearch.php
allows passing custom data to search engine
1.16.0SpecialStatsAddExtra
SpecialStatistics.php
Can be used to add extra statistic at the end of Special:Statistics
1.16.0SpecialUploadComplete
SpecialUpload.php
Called after successfully uploading a file from Special:Upload
1.6.0SpecialVersionExtensionTypes
SpecialVersion.php
called when generating the extensions credits, use this to change the tables headers
1.18.0SpecialWatchlistFilters
SpecialWatchlist.php
Called after building form options at Watchlist.
1.14.0SpecialWatchlistQuery
SpecialWatchlist.php
Called when building sql query for SpecialWatchlist
1.18.0TestCanonicalRedirect
Wiki.php
Called when about to force a redirect to a canonical URL for a title when we have no other parameters on the URL.
1.14.0TitleArrayFromResult
TitleArray.php
called when creating an TitleArray object from a database result
1.16.0TitleGetRestrictionTypes
Title.php
Allows to modify the types of protection that can be applied.
1.20.0TitleIsAlwaysKnown
Title.php
Allows overriding default behaviour for determining if a page exists.
1.19.0TitleIsCssOrJsPage
Title.php
Called when determining if a page is a CSS or JS page.
1.19.0TitleIsMovable
Title.php
Called when determining if it is possible to move a page.
1.19.0TitleIsWikitextPage
Title.php
Called when determining if a page is a wikitext or should be handled by seperate handler (via ArticleViewCustom).
1.4.0TitleMoveComplete
Title.php
Occurs whenever a request to move an article is completed
1.19.0TitleReadWhitelist
Title.php
Called at the end of read permissions checks, just before adding the default error message if nothing allows the user to read the page.
1.18.0UndeleteForm::showHistory
SpecialUndelete.php
Called in UndeleteForm::showHistory, after a PageArchive object has been created but before any further processing is done.
1.18.0UndeleteForm::showRevision
SpecialUndelete.php
Called in UndeleteForm::showRevision, after a PageArchive object has been created but before any further processing is done.
1.18.0UndeleteForm::undelete
SpecialUndelete.php
Called un UndeleteForm::undelete, after checking that the site is not in read-only mode, that the Title object is not null and after a PageArchive object has been constructed but before performing any further processing.
1.9.0UndeleteShowRevision
SpecialUndelete.php
called when showing a revision in Special:Undelete
1.17.0UnitTestsList
ExtensionsTestSuite.php
Add tests that should be run as part of the unit test suite.
1.4.0UnknownAction
Wiki.php
Used to add new query-string actions
1.4.0UnwatchArticle
Article.php
Occurs whenever the software receives a request to unwatch an article
1.4.0UnwatchArticleComplete
Article.php
Occurs after the unwatch article request has been processed
1.16.0UploadCreateFromRequest
UploadBase.php
When UploadBase::createFromRequest has been called.
1.6.4UploadComplete
SpecialUpload.php
Called when a file upload has completed.
1.16.0UploadFormInitDescriptor
SpecialUpload.php
Occurs after the descriptor for the upload form as been assembled.
1.16.0UploadFormSourceDescriptors
SpecialUpload.php
Occurs after the standard source inputs have been added to the descriptor.
1.9.0UploadForm:BeforeProcessing
SpecialUpload.php
Called just before the file data (for example description) are processed, so extensions have a chance to manipulate them.
1.9.0UploadForm:initial
SpecialUpload.php
Called just before the upload form is generated
1.6.0UploadVerification
SpecialUpload.php
Called when a file is uploaded, to allow extra file verification to take place (deprecated)
1.17.0UploadVerifyFile
SpecialUpload.php
Called when a file is uploaded, to allow extra file verification to take place (preferred)
1.18.0UserAddGroup
User.php
Called when adding a group to an user.
1.13.0UserArrayFromResult
UserArray.php
called when creating an UserArray object from a database result
1.6.0userCan
Title.php
To interrupt/advise the "user can do X to Y article" check
1.12.0UserCanSendEmail
User.php
To override User::canSendEmail() permission check
1.5.7UserClearNewTalkNotification
User.php
called when clearing the "You have new messages!" message
1.11.0UserEffectiveGroups
User.php
Called in User::getEffectiveGroups()
1.13.0UserGetAllRights
User.php
After calculating a list of all available rights
1.18.0UserGetDefaultOptions
User.php
Called after fetching the core default user options.
1.13.0UserGetEmail
User.php
Called when getting an user email address
1.13.0UserGetEmailAuthenticationTimestamp
User.php
Called when getting the timestamp of email authentification
1.11.0UserGetImplicitGroups
User.php
Called in User::getImplicitGroups()
1.18.0UserGetLanguageObject
User.php
Called when getting user's interface language object.
1.14.0UserGetReservedNames
User.php
Allows to modify $wgReservedUsernames at run time.
1.11.0UserGetRights
User.php
Called in User::getRights()
1.16.0UserIsBlockedFrom
User.php
Check if a user is blocked from a specific page (for specific block exemptions).
1.14.0UserIsBlockedGlobally
User.php
Check if user is blocked on all wikis.
1.14.0UserLoadAfterLoadFromSession
User.php
Called to authenticate users on external/environmental means; occurs after session is loaded
1.13.0UserLoadDefaults
User.php
Called when loading a default user
1.15.0UserLoadFromDatabase
User.php
Called when loading a user from the database
1.13.0UserLoadFromSession
User.php
Called to authenticate users on external/environmental means
1.15.0UserLoadOptions
User.php
Called when user options/preferences are being loaded from the database
1.27.0UserLoggedIn
AuthManager.php
Called after a user is logged in
1.4.0UserLoginComplete
SpecialUserlogin.php
Occurs after a user has successfully logged in
1.4.0UserLogout
SpecialUserlogin.php
Occurs when the software receives a request to log out
1.4.0UserLogoutComplete
SpecialUserlogin.php
Occurs after a user has successfully logged out
1.14.0User::mailPasswordInternal
SpecialUserlogin.php
Before creation and mailing of a user's new temporary password
1.24.0UserMailerChangeReturnPath
UserMailer.php
Called to generate a VERP return address when UserMailer sends an email, with a bounce handling extension.
1.18.0UserRemoveGroup
User.php
Called when removing a group from an user.
1.5.7UserRetrieveNewTalks
User.php
called when retrieving "You have new messages!" message(s)
1.6.0UserRights
SpecialUserrights.php
Called after a user's group memberships are changed
1.14.0UserrightsChangeableGroups
SpecialUserrights.php
Called after the list of groups that can be manipulated via Special:UserRights is populated, but before it is returned.
1.15.0UserSaveOptions
User.php
Called just before saving user preferences/options
1.13.0UserSaveSettings
User.php
Called when saving user settings
1.13.0UserSetCookies
User.php
Called when setting user cookies
1.13.0UserSetEmail
User.php
Called when changing user email address
1.13.0UserSetEmailAuthenticationTimestamp
User.php
Called when setting the timestamp of email authentification
1.8.0UserToggles
User.php
Called before returning "user toggle names"
1.19.0UserToolLinksEdit
Linker.php
Contribs | Block)"
1.18.0WantedPages::getQueryInfo
SpecialWantedPages.php
Called in WantedPagesPage::getQueryInfo(), can be used to alter the SQL query which gets the list of wanted pages.
1.15.0WantedPages::getSQL
SpecialWantedPages.php
Called in WantedPagesPage::getSQL(), can be used to alter the SQL query which gets the list of wanted pages DEPRECATED: Use WantedPages::getQueryInfo
1.4.0WatchArticle
Article.php
Occurs whenever the software receives a request to watch an article
1.4.0WatchArticleComplete
Article.php
Occurs after the watch article request has been processed
1.17.0WatchlistEditorBuildRemoveLine
WatchlistEditor.php
Occurs when building remove lines in Special:Watchlist/edit
1.19.0WebRequestPathInfoRouter
WebRequest.php
While building the PathRouter to parse the REQUEST_URI.
1.20.0wfShellWikiCmd
GlobalFunctions.php
Called when generating a shell-escaped command line string to run a maintenance script.
1.15.0WikiExporter::dumpStableQuery
Export.php
Get the SELECT query for "stable" revisions dumps
1.6.0wgQueryPages
QueryPage.php
called when initialising $wgQueryPages
1.16.0XmlDumpWriterOpenPage
Export.php
Called at the end of XmlDumpWriter::openPage, to allow extra metadata to be added.
1.16.0XmlDumpWriterWriteRevision
Export.php
Called at the end of a revision in an XML dump, to add extra metadata.
1.18.0XMPGetInfo
media/XMPInfo.php
Called when obtaining the list of XMP tags to extract.
1.18.0XMPGetResults
media/XMP.php
Called just before returning the results array of parsing xmp data.
Hooks grouped by version
To see hooks grouped by version go to the table above and click the arrow symbol in the version table header.
See also
[[Category:HooksTemplate:Translation]]
[[Category:Customization techniquesTemplate:Translation|Hooks]]
[[Category:MediaWiki hooksTemplate:Translation| ]]